FTRACKではPythonのAPIが公開されているので、より効率的にプロジェクトを運用することができます。
目次
インストール
① Pythonのダウンロード:
https://www.python.org/downloads/
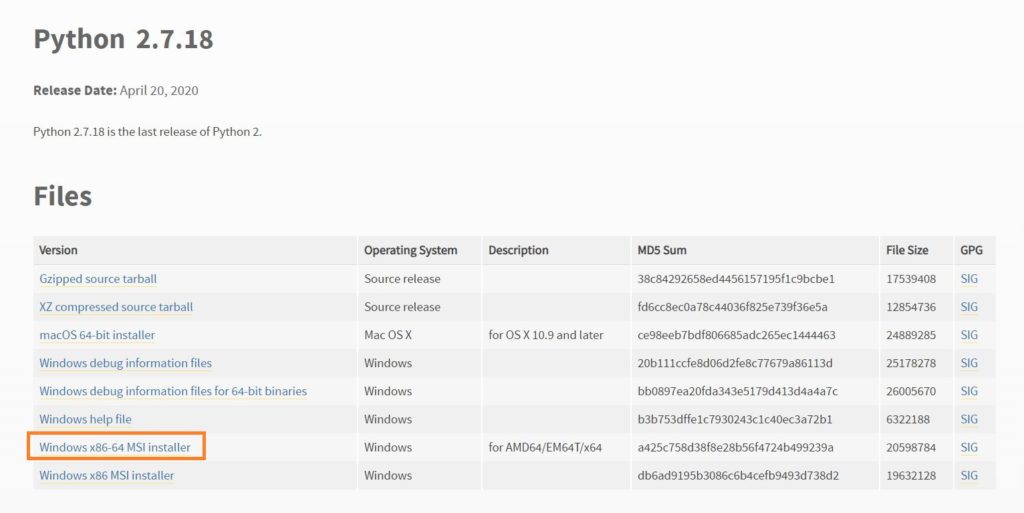
② pipを使用してAPIを取得
pipはコマンドプロンプトから使用できます。
スタートメニューの検索で「cmd.exe」と入力するか、
Win + R で"cmd"と入力。
pip install ftrack-python-api
変数 | 値 |
path | C:\Python27;C:\Python27\Scripts |
Tips
セッション
まずはftrack_apiのモジュールを読み込み、sessionで読み書きを行います。
import ftrack_api session = ftrack_api.Session( server_url='https://mycompany.ftrackapp.com', api_key='7545384e-a653-11e1-a82c-f22c11dd25eq', api_user='smartanimation' )
Querying
クエリは、Session.query()を使用して発行され、一致するエンティティのリストを返します。
http://ftrack-python-api.rtd.ftrack.com/en/stable/querying.html
サポートされている演算子のリスト
Operators | Description | Example |
= is | Exactly equal. | name is “martin” |
!= is_not | Not exactly equal. | name is_not “martin” |
> after greater_than | Greater than exclusive. | start after “2015-06-01” |
< before less_than | Less than exclusive. | end before “2015-06-01” |
>= | Greater than inclusive. | bid >= 10 |
<= | Less than inclusive. | bid <= 10 |
in | One of. | status.type.name in (“In Progress”, “Done”) |
not_in | Not one of. | status.name not_in (“Omitted”, “On Hold”) |
like | Matches pattern. | name like “%thrones” |
not_like | Does not match pattern. | name not_like “%thrones” |
has | Test scalar relationship. | author has (first_name is “Jane” and last_name is “Doe”) |
any | Test collection relationship. | metadata any (key=some_key and value=some_value) |
アクティブなプロジェクトを取得
session.query('Project where status is active')
タスクの取得
task = session.get('Task', '423ac382-e61d-4802-8914-dce20c92b740')
ユーザーの取得
user = session.query('User where username is "test_user"').one()
タスクに紐づいたアセットの取得
taskAssets = session.query('Asset where versions any (task_id is {0})'.format(task["id"]))
ユーザータスク
mytasks = session.query('Task where assignments any (resource_id={})'.format(user['id']))
バージョンのパブリッシュ
asset_parent = task['parent'] asset_type = session.query('AssetType where name is "Geometry"').one() asset = session.create('Asset', { 'name': 'My asset', 'type': asset_type, 'parent': asset_parent }) asset_version = session.create('AssetVersion', { 'asset': asset, 'task': task }) session.commit()
location = session.query('Location where name is "my-location"') asset_version.create_component( '/path/to/a/file.mov', data={ 'name': 'foobar' }, location=location )
Location
location = session.create('Location', dict(name='my.location')) session.commit()
ポイント
prefixにパスを記述して運用します。
import tempfile import ftrack_api.accessor.disk import ftrack_api.structure.id # Assign a disk accessor with *temporary* storage location.accessor = ftrack_api.accessor.disk.DiskAccessor( prefix=tempfile.mkdtemp() ) # Assign using ID structure. location.structure = ftrack_api.structure.id.IdStructure()
prefix="D:\publish"
のように指定することができます。
のように指定することができます。
ftrack_api.structure
- ftrack_api.structure.base
- ftrack_api.structure.id
- ftrack_api.structure.origin
- ftrack_api.structure.standard
standardを使用した例:
my_project/folder_a/folder_b/asset_name/v003/foo.jpg
structureを利用してフォルダのアップ場所やファイル名をカスタマイズできるよ!
プラグインパス
C:\Documents and Settings\<User>\Application Data\Local Settings\ftrack\ftrack-connect-plugins
デフォルト以外の場所:
FTRACK_CONNECT_PLUGIN_PATHを設定